Serial Module¶
Note
Usage¶
To easily display the Serial data received by the computer, tools like hterm, minicom or picocom can be used.
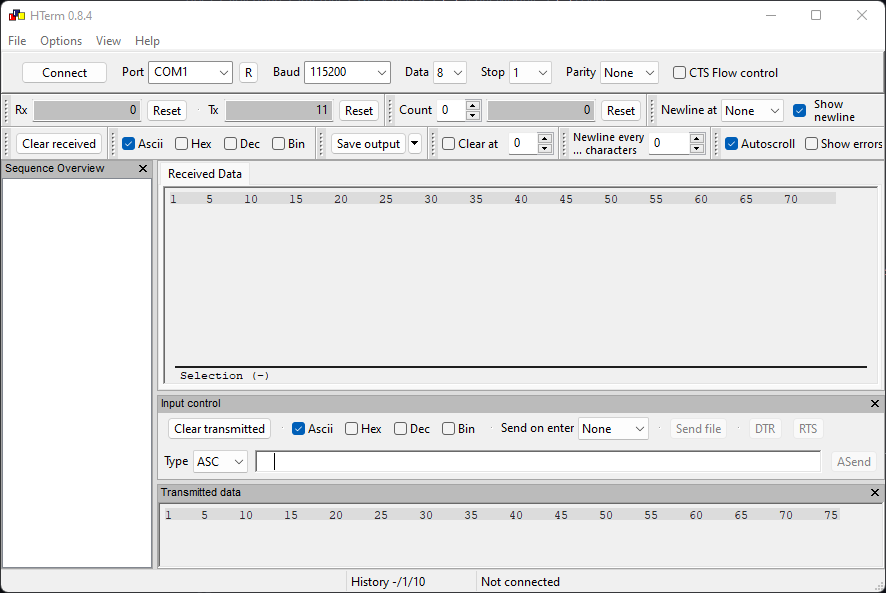
<pre><code>| To be able to talk to the Leguan board, make sure the following settings are correct: | | Baud Rate: 115200 | Data: 8 | Stop: 1 | Parity: None </code></pre>
Features¶
Important
The Serial / UART interface is in almost all cases used to transmit plain-text / ASCII data. Therefore all functions in this module accept NULL-Terminated C Strings. Do not pass binary data to them as these functions will assume they reached the end of the data when hitting a NULL byte!
Writing Strings¶
The following code can be used to transmit a string over the leguan’s virtual COM Port interface (routed through the usual USB-C port) to the computer.
// Send Hello World to computer. Try to do so for 100 ms before aborting operation
if (R_SUCCESS(SERIAL_Write("Hello World", 100))) {
// Successfully sent
}
-
result_t SERIAL_Write(const char *string, uint32_t timeout)¶
Writes a string to the UART interface.
- Parameters
string – String to write
timeout – Timeout
- Returns
Status result
Reading Strings¶
The following code can be used to receive a string over the leguan’s virtual COM Port interface (routed through the usual USB-C port) from the computer. Keep in mind that the buffer to receive data into needs to be one byte larger than the number of bytes you want to receive because it will include a NULL termination.
// Receives 10 characters from the computer. Last character will be a null termination
char buffer[10 + 1];
if (R_SUCCESS(SERIAL_Read(buffer, sizeof(buffer), 100))) {
// Successfully received
}
-
result_t SERIAL_Read(char *buffer, size_t size, uint32_t timeout)¶
Receives a string from the UART interface.
- Parameters
buffer – Buffer to write data to
size – Size of buffer
timeout – Timeout
- Returns
Status result